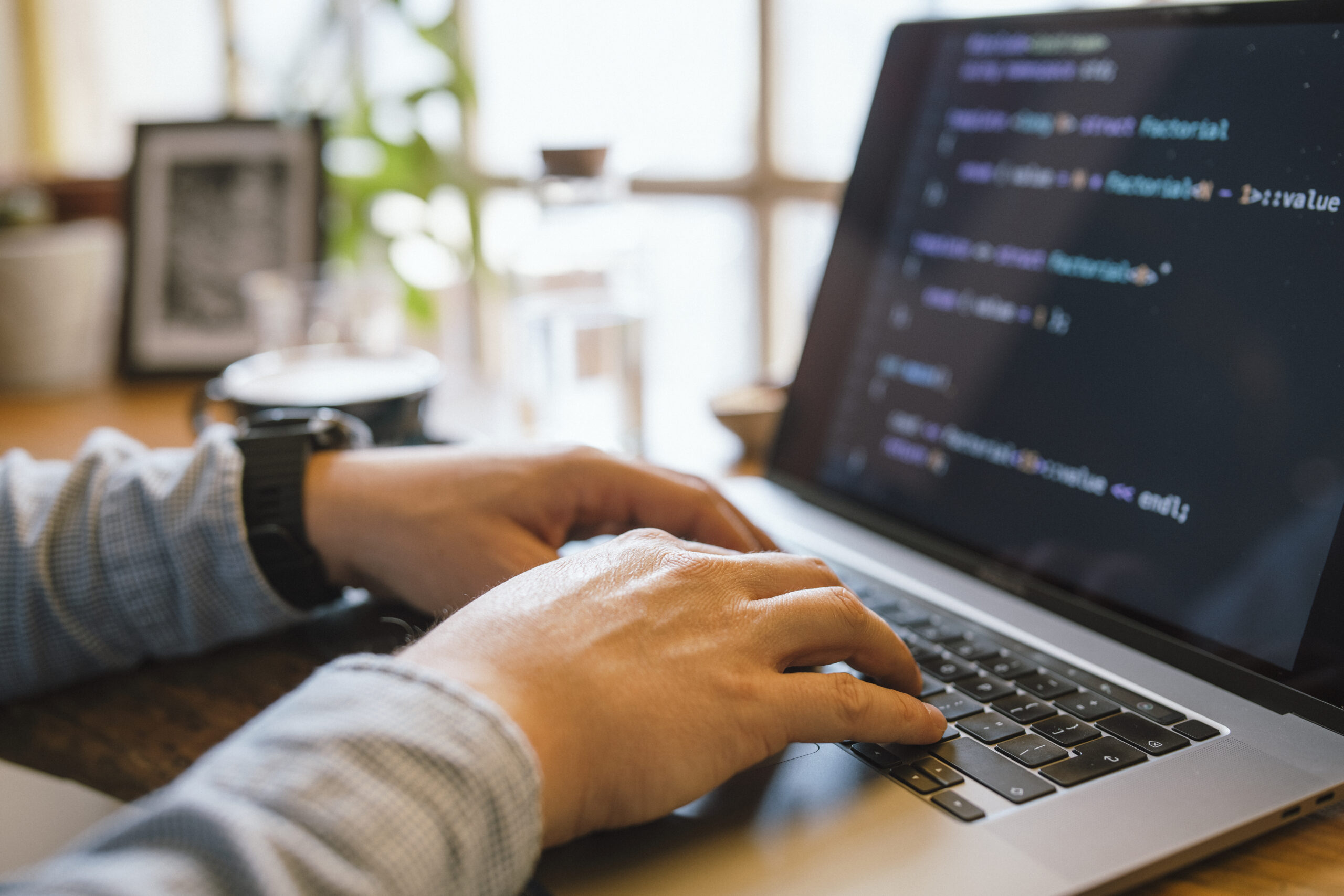
Debugging is One of the more important — nevertheless generally missed — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and considerably increase your productiveness. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although creating code is one Element of progress, being aware of the best way to interact with it correctly for the duration of execution is equally important. Modern-day growth environments come Geared up with effective debugging abilities — but several builders only scratch the floor of what these resources can perform.
Choose, by way of example, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to established breakpoints, inspect the worth of variables at runtime, stage via code line by line, and in some cases modify code within the fly. When utilized correctly, they Permit you to notice precisely how your code behaves all through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer resources, like Chrome DevTools, are indispensable for entrance-end developers. They allow you to inspect the DOM, observe community requests, see authentic-time overall performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of running processes and memory management. Mastering these tools might have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Edition Management devices like Git to understand code background, uncover the exact second bugs have been launched, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about producing an personal familiarity with your progress ecosystem so that when issues arise, you’re not lost at midnight. The better you realize your resources, the more time you are able to invest solving the actual problem instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Talk to inquiries like: What actions triggered the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the much easier it turns into to isolate the precise problems under which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, think about producing automated exams that replicate the sting instances or condition transitions associated. These tests not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, the issue could possibly be ecosystem-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination prospective fixes securely, and talk a lot more Obviously along with your crew or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Instead of looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, particularly when less than time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line range? What module or perform activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides real-time insights into how an application behaves, helping you understand what’s happening underneath the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what stage. Frequent logging amounts contain DEBUG, Information, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout development, INFO for normal gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for true issues, and Lethal if the program can’t carry on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and slow down your technique. Concentrate on vital functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it is a form of investigation. To efficiently establish and take care of bugs, developers should strategy the method similar to a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as you'll be able to with no jumping to conclusions. Use logs, check instances, and consumer studies to piece with each other a clear picture of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been built into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Try to recreate the problem in a very managed atmosphere. If you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay back near interest to smaller specifics. Bugs often cover in the the very least expected destinations—like a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely being familiar with it. Short term fixes may perhaps conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Other folks understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and become simpler at uncovering concealed difficulties in complex techniques.
Publish Checks
Writing tests is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early and also function a security Web that offers you assurance when making modifications in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Future, combine integration tests and end-to-conclusion exams into your workflow. These assist ensure that several areas of your application do the job collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several factors or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see the issue from the new standpoint.
If you're far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. Within this state, your Mind results in being fewer successful at challenge-fixing. A short walk, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate within the background.
Breaks also assistance avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it in fact causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught before with superior tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've acquired from the bug along with your peers is usually In particular effective. Regardless of whether it’s through a Slack concept, a short generate-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, a lot of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more click here able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and persistence — although the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.